1. Introduction
This portfolio documents my role and contributions to the project, Hallper. This project was undertaken as part of a requirement for the module CS2103T - Software Engineering, offered in the National University of Singapore (NUS). The main goal of the project is to enhance and add value to the application, AddressBook Level 4. Our team of five, decided to morph the AddressBook Level 4 into the application, Hallper.
About Hallper: Hallper is a desktop application providing administrative convenience. It is catered to the Junior Common Room Committee (JCRC) of the Halls of NUS, whose main responsibility is to organise and manage all student related activities in hall and look out for the welfare of the residents. Users interact with Hallper using a Command Line Interface (CLI), whilst a Graphic User Interface (GUI) implemented with JavaFX displays the relevant information to the user. Hallper is written in Java and contains approximately 60k lines of code (LoC). Integrated within Hallper is a range of features such as calendar, email and budgeting. Besides that, features such as erase, search and clear from the existing AddressBook Level 4 application have been enhanced.
My role and contributions: My contribution to the project is the implementation of the calendar feature and all its relevant commands. The commands that facilitate the calendar feature include creating calendars, adding events, deleting events and viewing calendars. My role in this project is to make sure that the milestones we set collectively are met on time. This includes reviewing the codes of my team members and doing acceptance testing (making sure the feature solves the problem it claims to solve).
2. Summary of contributions
This section lists the major and minor enhancements I have contributed to the project, Hallper, as well as miscellaneous contributions in other aspects of the project.
-
Major enhancement 1: added the ability to create calendars in Hallper
-
What it does: This feature allows the user (JCRC member) to create monthly calendars in Hallper.
-
Justification: This feature improves the product significantly because a JCRC member needs to keep track of the monthly events happening in hall, and a calendar is just the right tool. Being able to keep track of hall events in a calendar also allows the JCRC member to mitigate event date clashes with ease.
-
Highlights: Calendars are created in the file format
.ics
. This file format is chosen because it is compliant with the RFC 5545 format, which is recognised in the industry. Albeit the implementation of this feature requires an in-depth understanding of the external library iCal4j, this is not an issue as the library is widely used and well documented so new developers can easily pick up and extend the current features.
-
-
Major enhancement 2: added the ability to view calendars in Hallper
-
What it does: This feature allows the user (JCRC member) to view monthly calendars in Hallper by displaying the calendar on the GUI.
-
Justification: This feature improves the user experience as the user can observe changes made on the calendar dynamically. Besides that, the calendar is displayed alongside the command line interface (CLI), eliminating the need to switch between windows. In other words, the user can create, modify and view calendars in Hallper just by typing on the keyboard.
-
Highlights: The implementation of this feature requires an in-depth understanding of Java FXML API, JavaFX, XML, JFxtras and how they work together. It was challenging as it required changes to existing commands and interaction with the main window had to be correctly handled.
-
-
Minor enhancement: added add event and delete event commands that allow the user to modify calendar content.
-
Code contributed:
[ Functional code ]
[ Test code ]
[ RepoSense ] -
Other contributions:
-
Enhancements to existing features:
-
Wrote tests for calendar features to increase coverage from 54% to 66%
(Pull request: #119)
-
-
Documentation:
-
Community:
-
Tools:
-
3. Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Calendar
This section lists features related to managing the calendar in Hallper.
Creating calendar : create_calendar
Creates a calendar file in Hallper for updating of events.
Format: create_calendar month/MMM year/YYYY
Example:
-
create_calendar month/Feb year/2018
Creates a calendar for the month ofFeb
and year2018
and saves it as a.ics
file.
Adding all day event into calendar : add_all_day_event
Adds an all day event into the calendar.
Format: add_all_day_event month/MMM year/YYYY date/DD title/NAME OF EVENT
Example:
Before executing command:
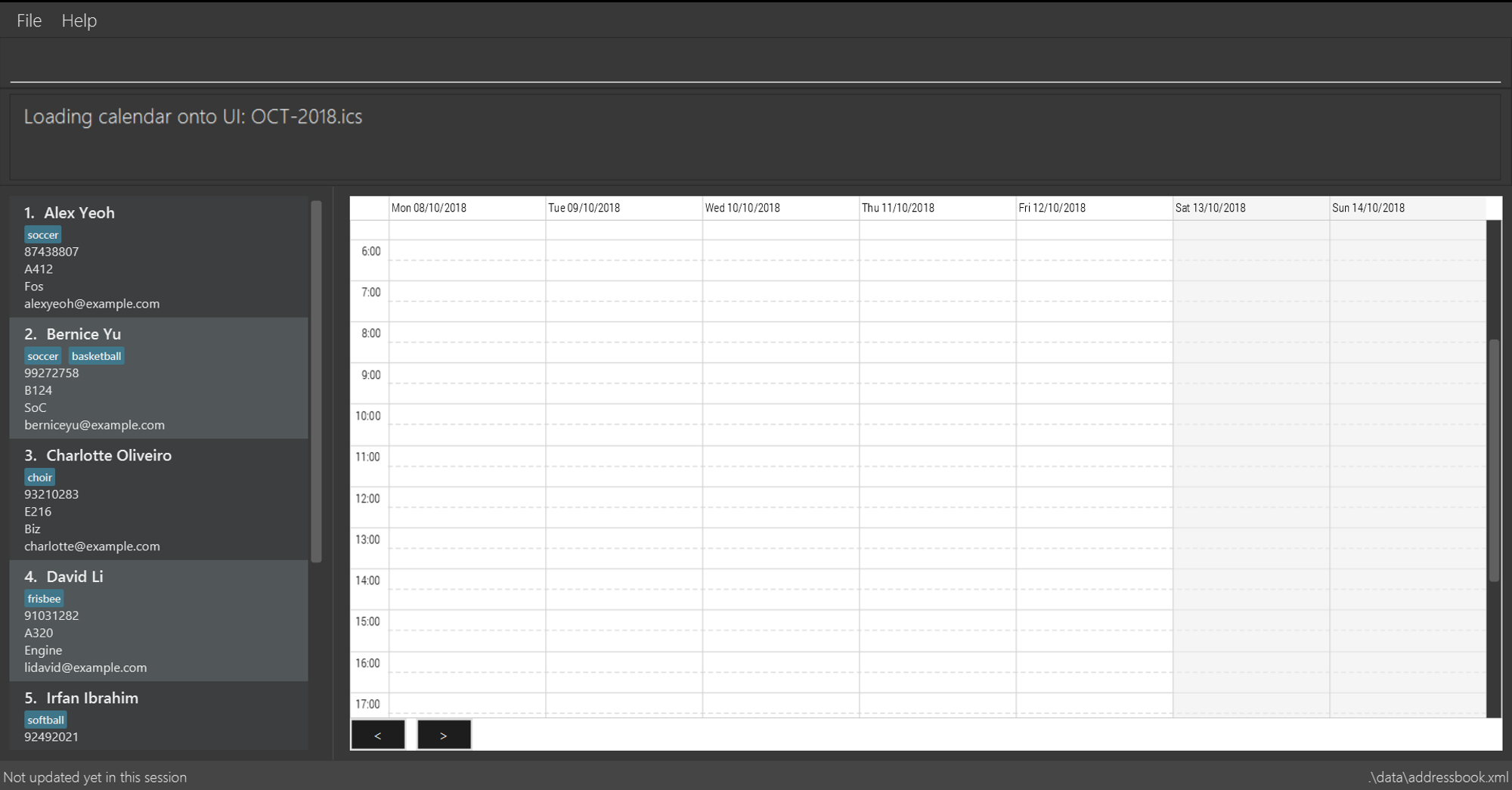
Figure 4.4.1.1: Screen before running add_all_day_event
on Hallper.
-
add_all_day_event month/Oct year/2018 date/08 title/Hall open day
Adds an all day event titledHall open day
into theOCT-2018.ics
calendar which happens on the8th
ofOct
.
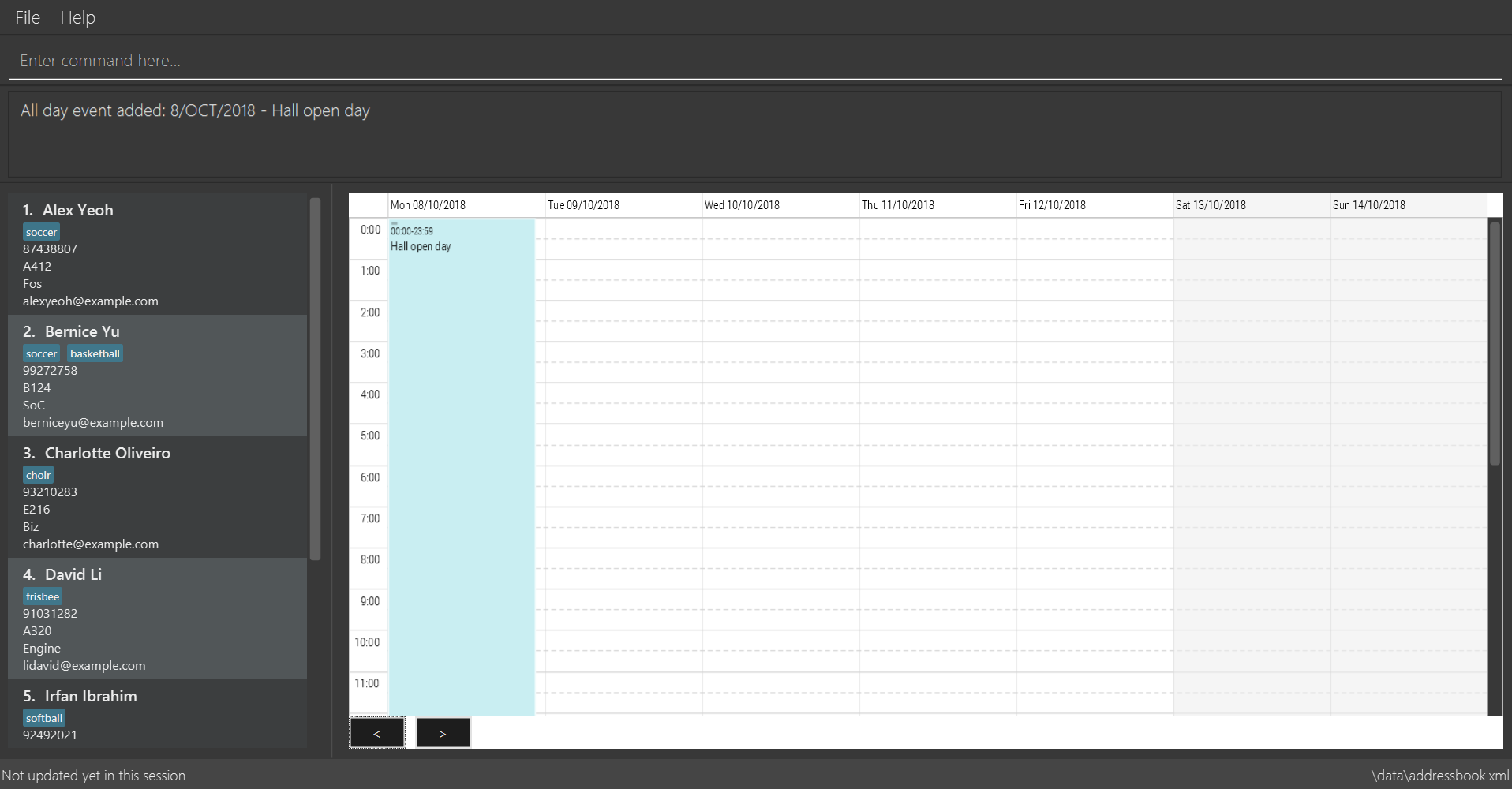
Figure 4.4.1.2: Screen after running add_all_day_event
on Hallper.
4. Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Calendar feature
Current Implementation
The calendar feature in Hallper is implemented using a third-party dependency, iCal4j.
It creates .ics
files and saves them onto the local computer. Calendars in Hallper are created as monthly
calendars, a Map<Year, Set<Month>>
in CalendarModel
keeps a record of existing calendars in Hallper.
It implements the following commands:
-
create_calendar
— Creates a monthly calendar and stores it in local memory. -
add_all_day_event
— Adds an all day event into the monthly calendar specified. -
add_event
— Adds an event of a specified time frame into the monthly calendar. -
delete_event
— Deletes an existing event in the monthly calendar. -
view_calendar
— Loads a monthly calendar from local memory onto the Hallper.
Create_Calendar Command
This command is facilitated by CalendarModel
and IcsCalendarStorage
.
It implements the following operations:
-
CalendarModel#createCalendar(Year year, Month month)
— Initializes a calendar object in theCalendarModel
. -
CalendarModel#isExistingCalendar(Year year, Month month)
— Checks if the calendar already exists in Hallper. -
IcsCalendarStorage#createCalendar(Calendar calendar, String calendarName)
— Saves the calendar passed fromCalendarModel
to the computer.
These operations are exposed in the Model
interface as Model#createCalendar(Year year, Month month)
, Model#isExistingCalendar(Year year, Month month)
and in the Storage
interface as Storage#createCalendar(Calendar calendar, String calendarName)
respectively.
Given below is an example usage scenario and how the create_calendar
command behaves at each step:
Step 1. The user executes the create_calendar
command by specifying the month and year.
The create_calendar
command then calls Model#isExistingCalendar(Year year, Month month)
, to check whether the
calendar already exists inside Hallper. If it exists, the command does nothing and reflects to the user that the
calendar already exists. Else, the command calls Model#createCalendar(Year year, Month month)
, initializing a calendar object inside CalendarModel
.
Step 2. Once the calendar object is initialized in the CalendarModel
, the ModelManager
raises a CalendarCreatedEvent
,
to indicate that a calendar object has been initialized in the CalendarModel
.
Step 3. The CalendarCreatedEvent
goes to the EventsCenter
, and is then handled by
StorageManager#handleCalendarCreatedEvent(CalendarCreatedEvent event)
, which then calls IcsCalendarStorage#createCalendar(Calendar calendar, String calendarName)
.
This saves the calendar to a specified local directory.
The following sequence diagram shows how the create_calendar operation works:
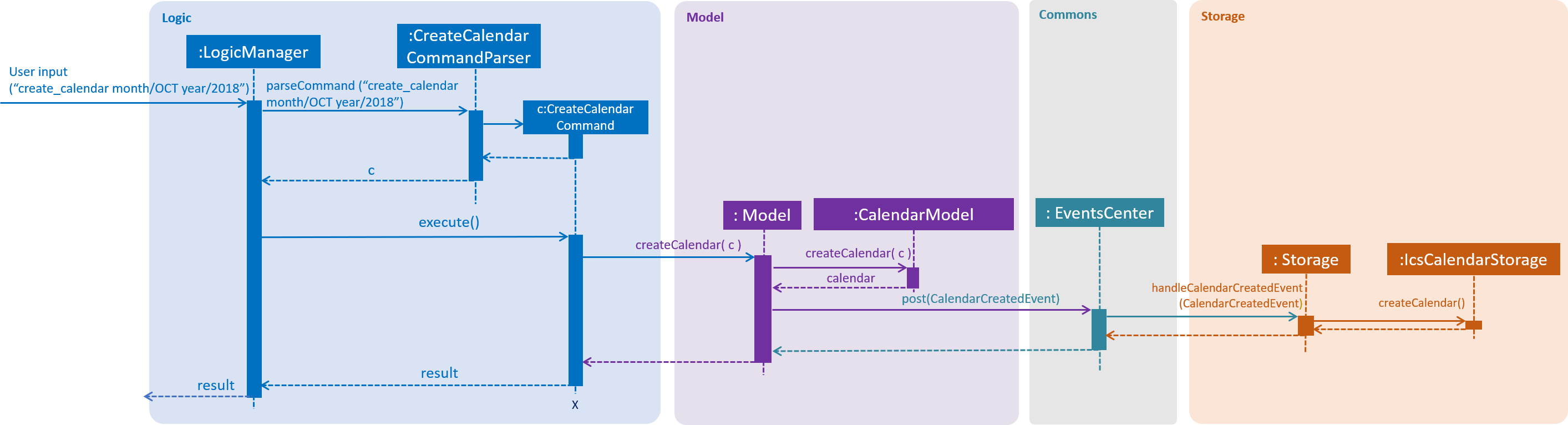
Figure 4.3.1.1: Sequence diagram for create_calendar command
Add Event Commands
The add_all_day_event
and add_event
command have many similarities, they differ only by their parameters and the number of checks called to verify the validity of the event to be added. Events in Hallper are created as VEvent objects as implemented in the iCal4j library.
Both commands are facilitated by ModelManager
, CalendarModel
and IcsCalendarStorage
. It implements the following operations:
-
ModelManager#loadCalendar(Year year, Month month)
— Raises aLoadCalendarEvent
. -
CalendarModel#createAllDayEvent(Year year, Month month, int date, String title)
— Creates an all day event object and saves it inside the loaded calendar inCalendarModel
. -
CalendarModel#createEvent(Year year, Month month, int startDate, int startHour, int startMin, int endDate, int endHour, int endMin, String title)
— Creates an event object with the specified time frame and saves it inside the loaded calendar inCalendarModel
. -
CalendarModel#loadCalendar(Year year, Month month)
— Loads the monthly calendar specified intoCalendarModel
. -
CalendarModel#isExistingCalendar(Year year, Month month)
— Checks if the calendar already exists in Hallper. -
CalendarModel#isValidDate(Year year, Month month, int date)
— Checks if the date is a valid date in accordance to the Gregorian calendar. -
CalendarModel#isValidTime(int hour, int min)
— Checks if the hour and minutes are valid in accordance to the 24 hour format. -
CalendarModel#isValidTimeFrame(int startDate, int startHour, int startMin, int endDate, int endHour, int endMin)
— Checks that the end date and time doesn’t occur before the start date and time. -
IcsCalendarStorage#loadCalendar(String calendarName)
— Loads the specified calendar from the local directory into local memory. -
IcsCalendarStorage#createCalendar(Calendar calendar, String calendarName)
— Saves the calendar passed fromCalendarModel
to the local directory.
These operations are exposed in the Model
and Storage
interface as :
-
Model#createAllDayEvent(Year year, Month month, int date, String title)
-
Model#createEvent(Year year, Month month, int startDate, int startHour, int startMin, int endDate, int endHour, int endMin, String title)
-
Model#loadCalendar(Year year, Month month)
-
Model#isExistingCalendar(Year year, Month month)
-
Model#isValidDate(Year year, Month month, int date)
-
Model#isValidTime(int hour, int min)
-
Model#isValidTimeFrame(int startDate, int startHour, int startMin, int endDate, int endHour, int endMin)
-
Storage#loadCalendar(String calendarName)
-
Storage#createCalendar(Calendar calendar, String calendarName)
Add_All_Day_Event Command
Given below is an example usage scenario and how the add_all_day_event
command behaves at each step:
Step 1. The user executes the add_all_day_event
command by specifying the month, year, date and title.
The add_all_day_event
command then calls Model#isExistingCalendar(Year year, Month month)
, Model#isValidDate(Year year, Month month, int date)
, to perform checks on whether the
request to create event is valid. If it fails any one of the checks, the command does nothing and reflects to the user that the request to create event is not valid.
Step 2. Else if the calendar hasn’t been loaded yet, Model
first calls CalendarModel#loadCalendar(Year year, Month month)
to load the calendar into CalendarModel
.
Step 3. Once the calendar object is loaded in the CalendarModel
, it then calls Model#createAllDayEvent(Year year, Month month, int date, String title)
, which calls CalendarModel#createAllDayEvent(Year year, Month month, int date, String title)
.
This creates an event object and loads it with all the relevant information.
Step 4. The ModelManager
then raises a AllDayEventAddedEvent
, to indicate an all day event has been created in the CalendarModel
.
Step 5. The AllDayEventAddedEvent
goes to the EventsCenter
, and is then handled by
StorageManager#handleAllDayEventAddedEvent(AllDayEventAddedEvent event)
, which then calls IcsCalendarStorage#createCalendar(Calendar calendar, String calendarName)
.
This saves the updated calendar back to the local directory.
The following sequence diagram shows how the add_all_day_event operation works:
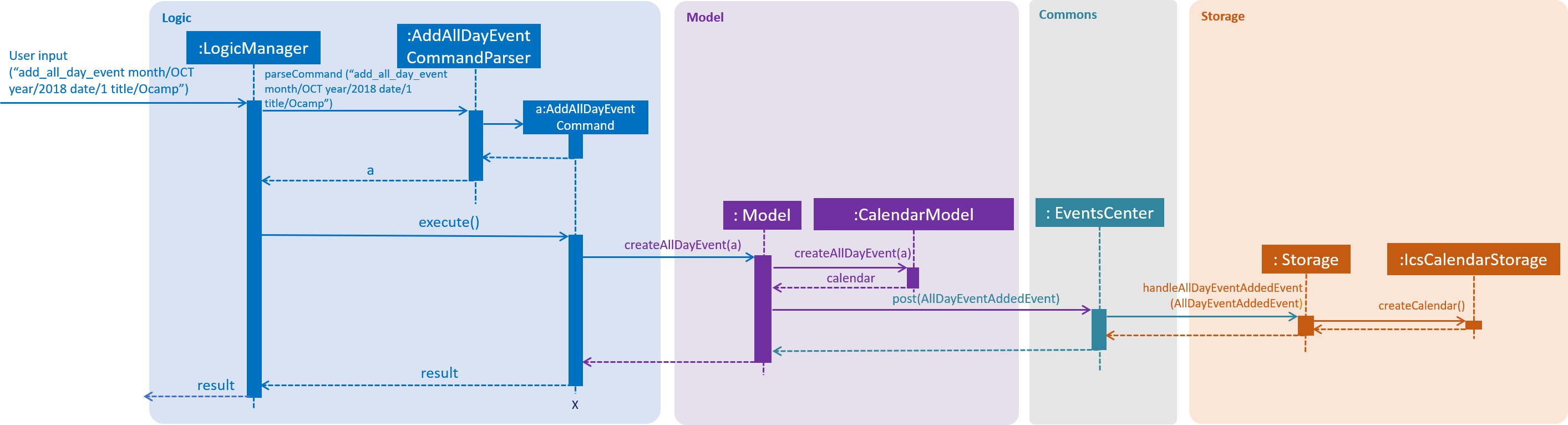
Figure 4.3.1.2: Sequence diagram for add_all_day_event command
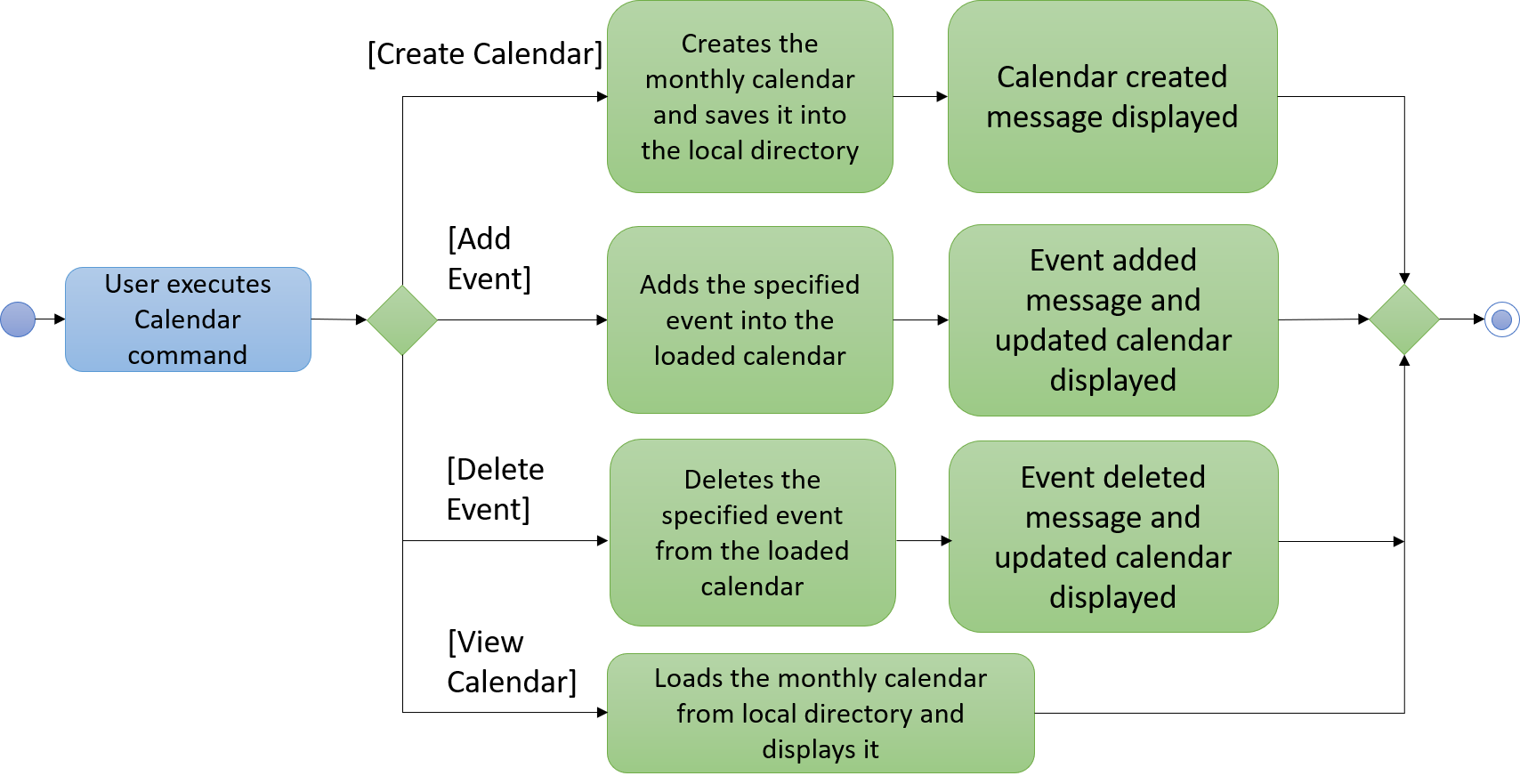
Figure 4.3.1.6: Activity diagram for calendar commands
Design Considerations
Aspect: File format
-
Pro: The
.ics
file format is compliant with the RFC 5545 format, which is industry recognised, and can be opened and viewed using many applications, including Microsoft Outlook, Google Calendar, and Apple Calendar. -
Con:
.ics
file alone is not very useful. User needs to import the events created into users' preferred calender application manually and consistently. More implementations will be required to make.ics
files useful in Hallper.
Aspect: Using iCal4j
iCal4j contains various methods that make creating, parsing and editing .ics
files convenient.
The library is widely used and easy to understand so any new developer can easily extend the current features.